Import Laravel Eloquent Project
This short tutorial demonstrates that in Skipper, import of an existing Laravel Eloquent project and the following model editing is simple and intuitive.
Hint: See the ORM Model basics for a detailed description of ORM elements (e.g. bundle, entity).
Import project
At first, we will import demo project to Laravel Eloquent Skipper project (Skipper supports only database import for Eloquent projects). To do this, select Import External Model from File menu and follow the steps shown in the pictures below.
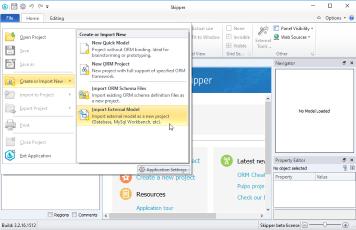
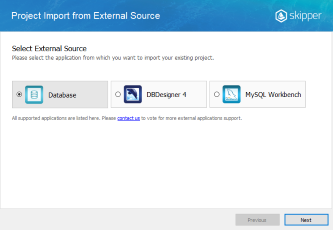
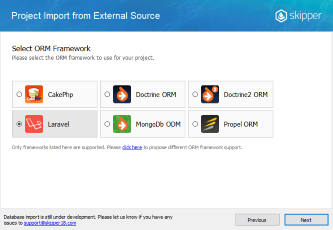
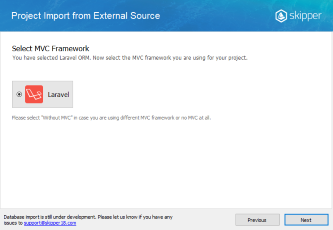
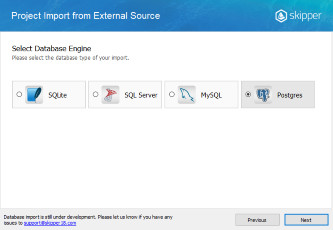
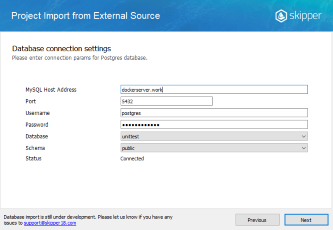
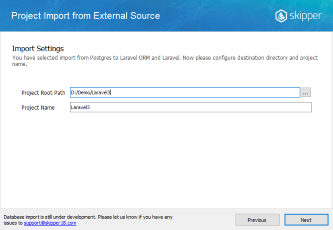
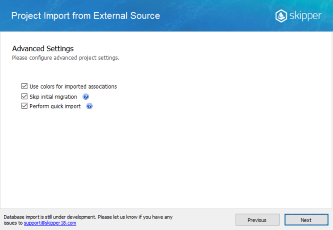
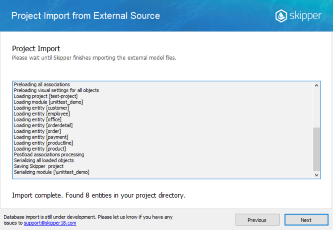
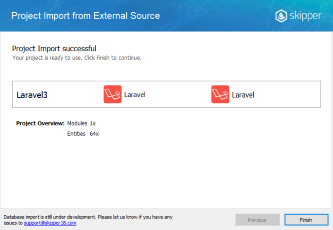
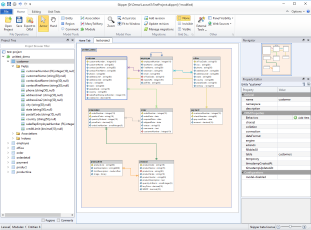
The last screen visually represents Demo project imported to Skipper and arranged automatically by import algorithm. It is a good starting point to work with your model.
Arrange visual model
Although the imported model is automatically arranged, it is a good idea to reposition entities inside the bundles to exactly fit the model logic. Also, you can change colors of bundles according to your preferences.
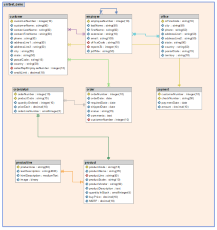
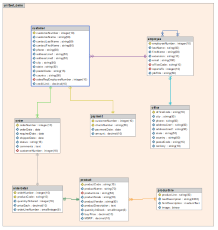
Extend project model
Now we have nice visual model. Let’s extend it to store categories for product entities. We add Category entity, configure new fields and create association between Category and Product entity.
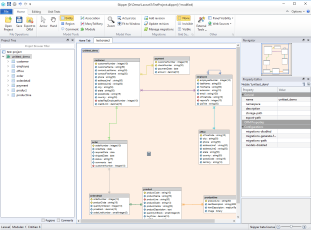
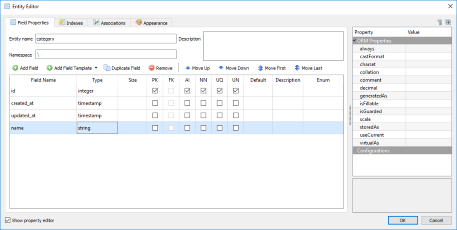
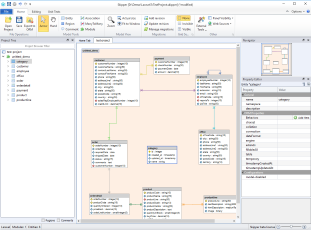
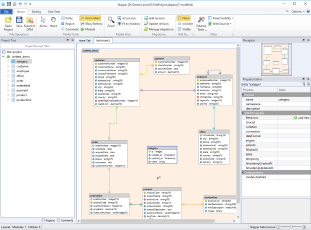
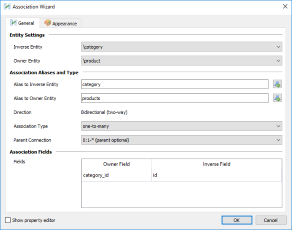
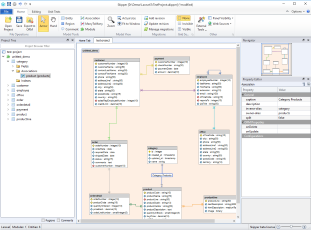
Export model
When we finished the application model editing, the next step is to export it to Laravel Eloquent model migrations, model base classes and derived model classes. Model classes are exported in accordance with their namespaces to corresponding directories (based on PSR-4). Migrations are exported to \database\migrations
directory which can be changed/configured for each module via ORM Properties.
Skipper exports base model object and derived model object for each Laravel Eloquent entity and one migration file for each defined migration in the application. Base model objects and migrations are updated during every export, but derived model objects are exported only once because they serves for defining user logic.
Before the first export we create our initial migration (revision), as shown in picture 2 below this section. After that, we export the whole project to a target directory. In our case, the project will export four schema model base classes, four derived classes and one migration file.
Tip: Each bundle might have its own export path which is relative to a project root directory.
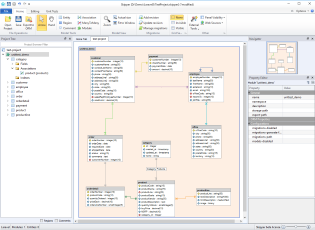
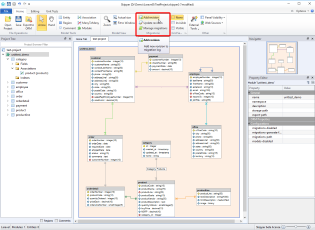
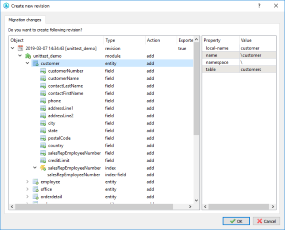
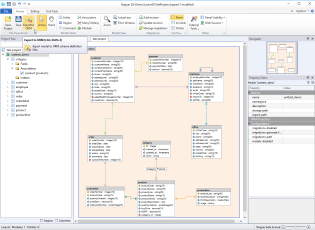
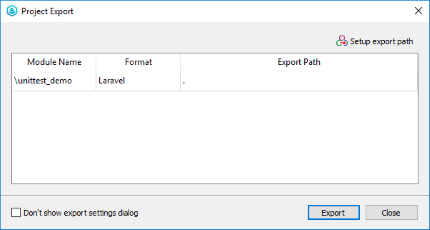
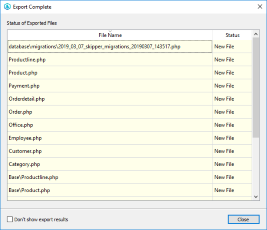
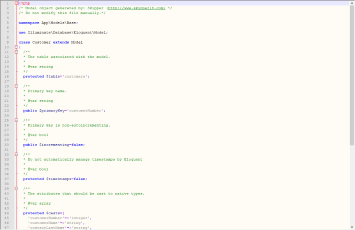
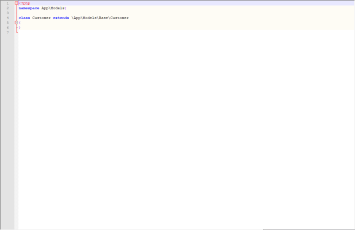
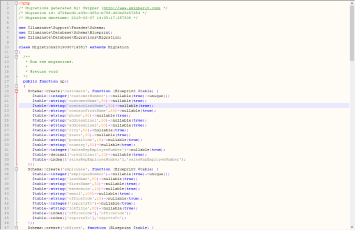
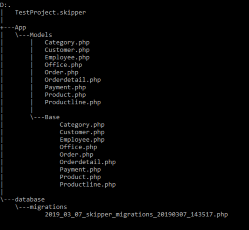
And here is a full example of Customer entity. As you can see everything is well formatted and defined by Laravel Eloquent coding rules.
Base class:
<?php
/* Model object generated by: Skipper (http://www.skipper18.com) */
/* Do not modify this file manually.*/
namespace App\Models\Base;
use Illuminate\Database\Eloquent\Model;
class Customer extends Model
{
/**
* The table associated with the model.
*
* @var string
*/
protected $table='customers';
/**
* Primary key name.
*
* @var string
*/
public $primaryKey='customerNumber';
/**
* Primary key is non-autoincrementing.
*
* @var bool
*/
public $incrementing=false;
/**
* Do not automatically manage timestamps by Eloquent
*
* @var bool
*/
protected $timestamps=false;
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts=[
'customerNumber'=>'integer',
'customerName'=>'string',
'contactLastName'=>'string',
'contactFirstName'=>'string',
'phone'=>'string',
'addressLine1'=>'string',
'addressLine2'=>'string',
'city'=>'string',
'state'=>'string',
'postalCode'=>'string',
'country'=>'string',
'salesRepEmployeeNumber'=>'integer',
'creditLimit'=>'decimal'
];
public function employees()
{
return $this->belongsTo('\App\Models\employee','salesRepEmployeeNumber','employeeNumber');
}
public function orders()
{
return $this->hasMany('\App\Models\order','customerNumber','customerNumber');
}
public function payments()
{
return $this->hasOne('\App\Models\payment','customerNumber','customerNumber');
}
}
Derived class:
<?php
namespace App\Models;
class Customer extends \App\Models\Base\Customer
{
}
Migrations file:
<?php
/* Migrations generated by: Skipper (http://www.skipper18.com) */
/* Migration id: d726acd0-a33c-465c-b79f-d00b25e57654 */
/* Migration datetime: 2019-03-07 14:35:17.257308 */
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class Migrations20190307143517 extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('customers', function (Blueprint $table) {
$table->integer('customerNumber')->nullable(true)->unique();
$table->string('customerName',50)->nullable(true);
$table->string('contactLastName',50)->nullable(true);
$table->string('contactFirstName',50)->nullable(true);
$table->string('phone',50)->nullable(true);
$table->string('addressLine1',50)->nullable(true);
$table->string('addressLine2',50)->nullable(true);
$table->string('city',50)->nullable(true);
$table->string('state',50)->nullable(true);
$table->string('postalCode',15)->nullable(true);
$table->string('country',50)->nullable(true);
$table->integer('salesRepEmployeeNumber')->nullable(true);
$table->decimal('creditLimit',10)->nullable(true);
$table->index(['salesRepEmployeeNumber'],'salesRepEmployeeNumber');
});
Schema::create('employees', function (Blueprint $table) {
$table->integer('employeeNumber')->nullable(true)->unique();
$table->string('lastName',50)->nullable(true);
$table->string('firstName',50)->nullable(true);
$table->string('extension',10)->nullable(true);
$table->string('email',100)->nullable(true);
$table->string('officeCode',10)->nullable(true);
$table->integer('reportsTo')->nullable(true);
$table->string('jobTitle',50)->nullable(true);
$table->index(['officeCode'],'officeCode');
$table->index(['reportsTo'],'reportsTo');
});
Schema::create('offices', function (Blueprint $table) {
$table->string('officeCode',10)->nullable(true)->unique();
$table->string('city',50)->nullable(true);
$table->string('phone',50)->nullable(true);
$table->string('addressLine1',50)->nullable(true);
$table->string('addressLine2',50)->nullable(true);
$table->string('state',50)->nullable(true);
$table->string('country',50)->nullable(true);
$table->string('postalCode',15)->nullable(true);
$table->string('territory',10)->nullable(true);
});
Schema::create('orderdetails', function (Blueprint $table) {
$table->integer('orderNumber')->nullable(true)->unique();
$table->string('productCode',15)->nullable(true)->unique();
$table->integer('quantityOrdered')->nullable(true);
$table->decimal('priceEach',10)->nullable(true);
$table->smallInteger('orderLineNumber')->nullable(true);
$table->index(['productCode'],'productCode');
$table->primary(['orderNumber','productCode']);
});
Schema::create('orders', function (Blueprint $table) {
$table->integer('orderNumber')->nullable(true)->unique();
$table->date('orderDate')->nullable(true);
$table->date('requiredDate')->nullable(true);
$table->date('shippedDate')->nullable(true);
$table->string('status',15)->nullable(true);
$table->text('comments')->nullable(true);
$table->integer('customerNumber')->nullable(true);
$table->index(['customerNumber'],'customerNumber');
});
Schema::create('payments', function (Blueprint $table) {
$table->integer('customerNumber')->nullable(true)->unique();
$table->string('checkNumber',50)->nullable(true)->unique();
$table->date('paymentDate')->nullable(true);
$table->decimal('amount',10)->nullable(true);
$table->primary(['customerNumber','checkNumber']);
});
Schema::create('productlines', function (Blueprint $table) {
$table->string('productLine',50)->nullable(true)->unique();
$table->string('textDescription',4000)->nullable(true);
$table->mediumText('htmlDescription')->nullable(true);
$table->binary('image')->nullable(true);
});
Schema::create('products', function (Blueprint $table) {
$table->string('productCode',15)->nullable(true)->unique();
$table->string('productName',70)->nullable(true);
$table->string('productLine',50)->nullable(true);
$table->string('productScale',10)->nullable(true);
$table->string('productVendor',50)->nullable(true);
$table->text('productDescription')->nullable(true);
$table->smallInteger('quantityInStock')->nullable(true);
$table->decimal('buyPrice',10)->nullable(true);
$table->decimal('MSRP',10)->nullable(true);
$table->integer('category_id')->nullable(true)->unsigned();
$table->index(['productLine'],'productLine');
});
Schema::create('categories', function (Blueprint $table) {
$table->integer('id')->autoIncrement()->unsigned();
$table->timestamp('created_at')->nullable(true);
$table->timestamp('updated_at')->nullable(true);
$table->string('name')->nullable(true);
});
Schema::table('customers', function (Blueprint $table) {
$table->foreign('salesRepEmployeeNumber')->references('employeeNumber')->on('employees');
});
Schema::table('employees', function (Blueprint $table) {
$table->foreign('reportsTo')->references('employeeNumber')->on('employees');
});
Schema::table('employees', function (Blueprint $table) {
$table->foreign('officeCode')->references('officeCode')->on('offices');
});
Schema::table('orderdetails', function (Blueprint $table) {
$table->foreign('orderNumber')->references('orderNumber')->on('orders');
});
Schema::table('orderdetails', function (Blueprint $table) {
$table->foreign('productCode')->references('productCode')->on('products');
});
Schema::table('orders', function (Blueprint $table) {
$table->foreign('customerNumber')->references('customerNumber')->on('customers');
});
Schema::table('payments', function (Blueprint $table) {
$table->foreign('customerNumber')->references('customerNumber')->on('customers');
});
Schema::table('products', function (Blueprint $table) {
$table->foreign('productLine')->references('productLine')->on('productlines');
});
Schema::table('products', function (Blueprint $table) {
$table->foreign('category_id')->references('id')->on('categories');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('products', function (Blueprint $table) {
$table->dropForeign(['category_id']);
});
Schema::table('products', function (Blueprint $table) {
$table->dropForeign(['productLine']);
});
Schema::table('payments', function (Blueprint $table) {
$table->dropForeign(['customerNumber']);
});
Schema::table('orders', function (Blueprint $table) {
$table->dropForeign(['customerNumber']);
});
Schema::table('orderdetails', function (Blueprint $table) {
$table->dropForeign(['productCode']);
});
Schema::table('orderdetails', function (Blueprint $table) {
$table->dropForeign(['orderNumber']);
});
Schema::table('employees', function (Blueprint $table) {
$table->dropForeign(['officeCode']);
});
Schema::table('employees', function (Blueprint $table) {
$table->dropForeign(['reportsTo']);
});
Schema::table('customers', function (Blueprint $table) {
$table->dropForeign(['salesRepEmployeeNumber']);
});
Schema::dropIfExists('categories');
Schema::dropIfExists('products');
Schema::dropIfExists('productlines');
Schema::dropIfExists('payments');
Schema::dropIfExists('orders');
Schema::dropIfExists('orderdetails');
Schema::dropIfExists('offices');
Schema::dropIfExists('employees');
Schema::dropIfExists('customers');
}
}
Next time you will need to make further changes or adjust the model, just open Skipper file and edit your project entities. Then again export the project to model classes and migrations.
Conclusion
As you can see above, import, editing and export to schema definition files is a five minute work with Skipper (ten if you are doing it for the first time). Now compare it with the effort of doing such task manually. As you have just discovered, Skipper dramatically saves your time and helps you work with schema definitions in much more enjoyable way.